· 3 min read
Using Anko to run background tasks with Kotlin in Android (KAD 09)
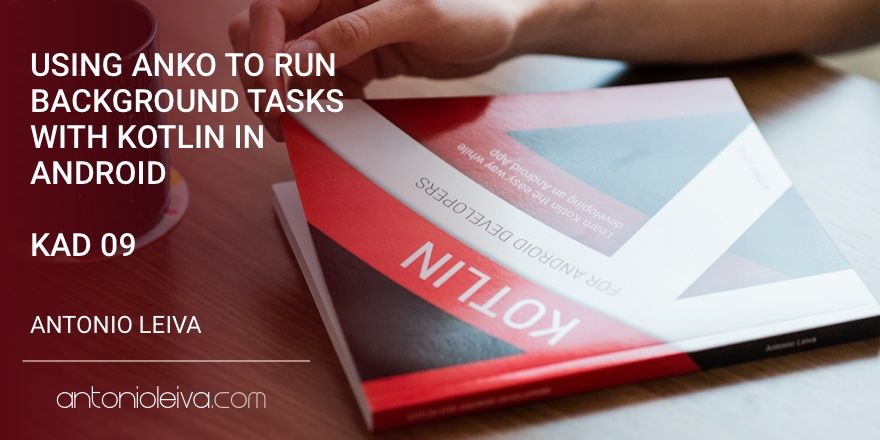
Anko is an Android library written in Kotlin by Jetbrains, that can be used for a lot of different stuff. Its main feature is to create views by code using a DSL.
Although this can be very interesting, the truth is that I got along very well with XMLs for so long. So I haven’t tried this feature much.
But it does have other very useful features, and the one I tell you today is quite cool.
Anko used to execute background tasks
The issue of getting out of the main thread to execute operations that can block is very recurrent in Android.
There are thousands of alternatives, from several offered by the framework (such as AsyncTask or Loader) to libraries. Some even use RxJava for this.
All are valid options, and all have their pros and cons.
But most of them are very complex just to achieve the simple goal of getting out of the main thread to do some heavy task.
Anko takes advantage of Kotlin power to offer an easy and light solution to free the main thread from long-running tasks.
Disclaimer: This article just wants to show what the power of Kotlin can allow. As of today, I wouldn’t use this mechanism for background tasks, I prefer Kotlin Coroutines instead.
Add the dependency to your project
The first thing is to include the dependency.
As Anko does many things, the size of the library got out of hand. So there came a time when they decided to break it.
For this example, you only need to add the following import:
implementation 'org.jetbrains.anko:anko-common:0.9'
Run the task in a background thread
If you remember, in a previous article we implemented a pretty basic doAsync
, which could run background operations. Anko is able to use the execution context to do one thing or the other. We’ll see an example later.
For now, the resulting code is very similar:
doAsync {
var result = runLongTask()
}
But how do we get back to the main thread?
Returning to the main thread
It is also very simple. You just have to write a uiThread
block inside the doAsync
, and this will run on the main thread.
doAsync {
var result = runLongTask()
uiThread {
toast(result)
}
}
toast()
is another useful function provided by Anko, which simplifies the way we display toasts in our App.
But the important part is that of uiThread
. This runs on the main thread.
And you know what? If the doAsync
was called by an Activity
, the code of uiThread
will not be executed if the Activity
is dying (isFinishing
returns true
). This way, we avoid a recurrent error that usually happens with the AsyncTask
or any other callback that does not pay attention to the activity lifecycle.
Conclusion
As you can see, Anko provides a set of utilities that will simplify our life when writing Android Apps. There are many others, such as dialog creation or database management, which we may look at in the following articles.
If all this passionate you as to me, I encourage you to sign up for my free training where I will tell you everything you need to learn about how to create your own Android Apps in Kotlin from scratch.