· 4 min read
View Binding: The Definitive way to access views on Android
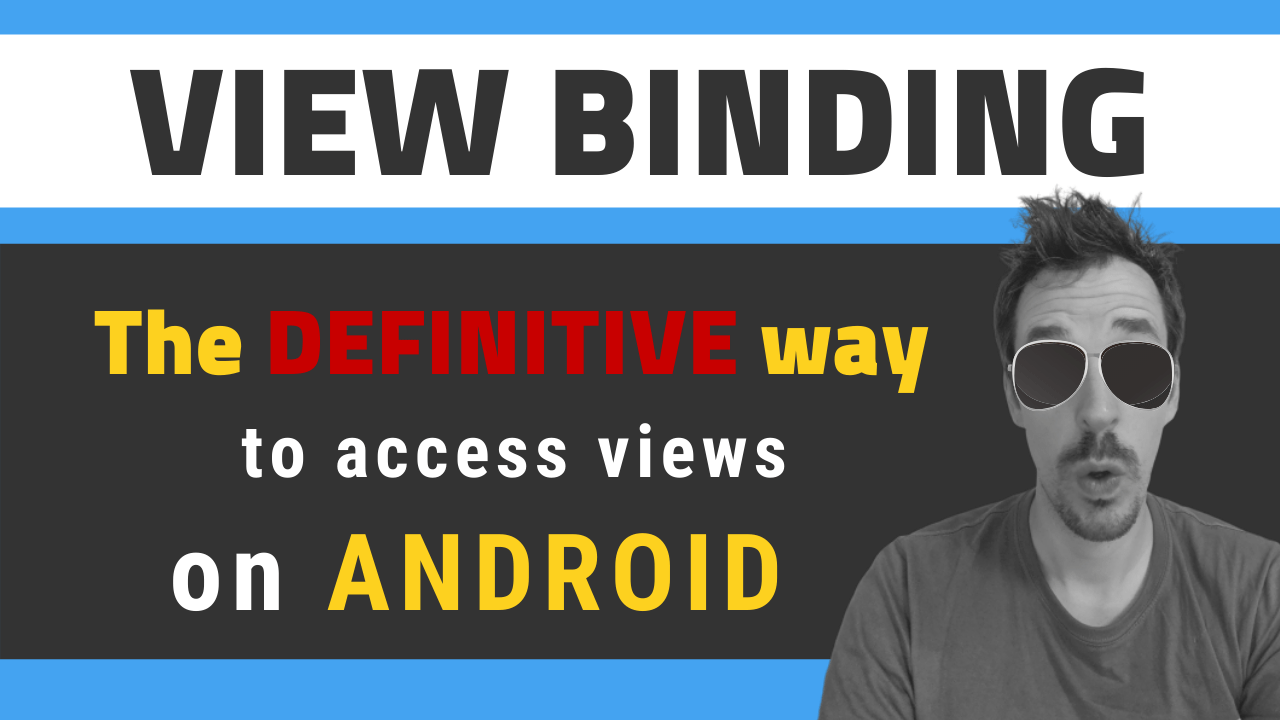
View Binding is a new view access mechanism that was released in conjunction with Android Studio version 3.6.
Historically, there have been many ways to access views in an XML, as the original form, using findViewById
, was not particularly comfortable.
If we had to highlight two, these would be ButterKnife, and the Kotlin Android Extensions sythentics.
But with View Binding we go a little further, and it has several advantages that make it the most interesting option today.
View Binding: What is it?
For some time, a library known as Data Binding has been in Android development.
This library allows you to access the views in a very simple way, linking variables of our Kotlin or Java code with the XML components.
This is achieved thanks to the fact that it enables the possibility of adding code within the XMLs.
Data Binding graph extracted from code-labs.io
This solution is very interesting, but perhaps too invasive for some developers, who see their XMLs begin to fill with code. Well used is very interesting, but it is kind of easy to misuse it.
In addition, XMLs need to be modified where they are used, and it increases compilation times noticeably.
But there is a subset of Data Binding that is very easy to use and greatly simplifies life.
When a layout is inflated, it creates a Binding
object, which contains all the XML views casted to the correct type, also taking into account the possible nullity of each view.
This greatly simplifies the work, since in a single line we can retrieve all the views and have them ready for use.
With View Binding, they have extracted this functionality, optimized compile times, and saved us the need to modify the XML in order to use it.
In literally 2 lines of code, you will have your XML ready to be converted into binding objects.
View Binding Configuration
Getting started with View Binding is as easy as adding a line of code to the build.gradle
android {
viewBinding.enabled = true
}
How to use View Binding in an Activity
All you need to do is modify the way you inflate your layouts. Instead of calling setContentView
with the identifier of the layout, you will do it by passing it the view that you have previously inflated with View Binding, like this:
val binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.root
contiene el root del layout que ha sido previamente inflado. Ahora solo necesitas acceder a las propiedades del objeto para usar sus vistas:
binding.button.setOnClickListener {
...
}
And how to use it in an Adapter?
Here are at least a couple of ways to do it:
- Using the
inflate
method in the onCreateViewHolder and storing the binding object - Inflating the layout the classic way, and using the
bind
method in the ViewHolder to make it available there.
I personally like the second way more, because there is no need to worry about passing the object from one point to another of the adapter. The binding is created right where it is needed.
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val v = parent.inflate(R.layout.view_media_item, false)
return ViewHolder(
v,
listener
)
}
class ViewHolder(view: View, private val listener: MediaListener) :
RecyclerView.ViewHolder(view) {
private val binding = ViewMediaItemBinding.bind(view)
}
From that point on, it can already be used exactly as we did in the Activity.
Why should you use it versus other alternatives?
The truth is that the rest of the options are still valid, but with some nuances.
ButterKnife, the most popular one, is deprecated in favor of View Binding.
On the other hand, synthetics are a bit “darker”, since we don’t have much control over how they work, and also leave the decision on the nullity of each view to the programmer. They don’t assist in any way regarding this.
This table is taken from the article on the Android Developers blog:
Should I then migrate all my code to View Binding?
As I usually say, that something new appears doesn’t mean that we should migrate all our code.
In this case, being such a light and easy to integrate feature, it would be safe to think of using it on new screens, and even refactor those that you must modify for some reason.
In any case, as everything, this is optional and you should only do it if you feel comfortable with it.
Conclusion
I hope that this article has helped you to clarify some questions regarding View Binding, and that you are encouraged to try it, because it can simplify your day to day.
If you are interested, you can see a sample code about View Binding on my GitHub.
Also, I’ve posted a cheatsheet on View Binding on Instagram.
And I have a video on YouTube:
For any questions, I will read you in the comments!