· 3 min read
Using "when" expression in Kotlin: The "switch" with super powers (KAD 13)
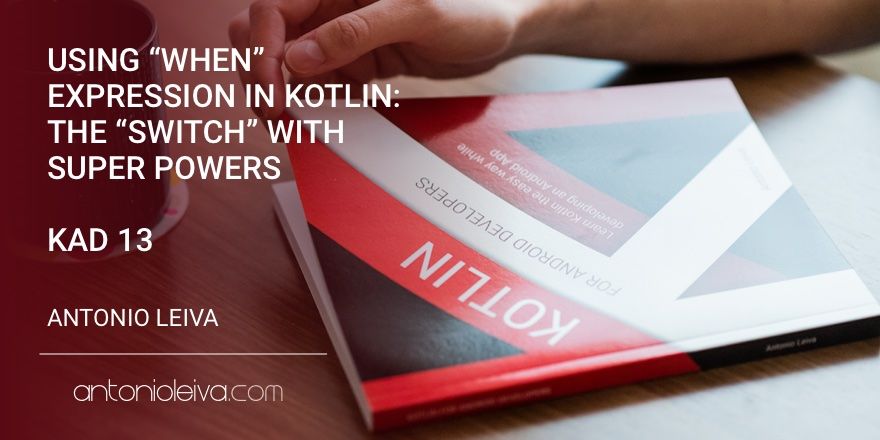
The switch
expression in Java, and especially in Java 6, are extremely limited. Apart from a very short amount of types, it can not be used for anything else.
But, however, when
expressions in Kotlin can do everything you can do with a switch
and much more.
Actually, with when
you can substitute the most complex if/else
you can have in your code.
Would you like to start today to take the next step? I recommend that you sign up to my free training here.
when expression in Kotlin
For starters, you can use it as a regular switch
. Imagine that, for example, you have a view and want to display a toast based on its visibility.
You can do:
when(view.visibility){
View.VISIBLE -> toast("visible")
View.INVISIBLE -> toast("invisible")
else -> toast("gone")
}
In when
, else
is the same as default
for switch
. You give a solution for the rest of the alternatives you’re not covering in the expression.
But when
has some other extra features that make it really powerful:
Auto-casting
If you check something in the left side (for instance, that an object is an instance of an specific type), you’ll get the cast in the right side for free:
when (view) {
is TextView -> toast(view.text)
is RecyclerView -> toast("Item count = ${view.adapter.itemCount}")
is SearchView -> toast("Current query: ${view.query}")
else -> toast("View type not supported")
}
Besides checking a type, when
can check for instance if an element is inside a range or a list, by using the reserved word in
.
when without arguments
With this option, we can check basically anything we want in the left side of the when
condition.
val res = when {
x in 1..10 -> "cheap"
s.contains("hello") -> "it's a welcome!"
v is ViewGroup -> "child count: ${v.getChildCount()}"
else -> ""
}
As when
is an expression, it can return a value that can be stored in a variable.
An example applied to Android
The above examples are very simple and far from having any real use.
But an example that I like a lot is to consume the answer in an onOptionsItemSelected()
.
override fun onOptionsItemSelected(item: MenuItem) = when (item.itemId) {
R.id.home -> consume { navigateToHome() }
R.id.search -> consume { MenuItemCompat.expandActionView(item) }
R.id.settings -> consume { navigateToSettings() }
else -> super.onOptionsItemSelected(item)
}
The consume
function is a very simple one that executes the operation and returns true
. I find it very useful for the methods of the Android framework that need to indicate if they have consumed the result.
The code involved is very simple:
inline fun consume(f: () -> Unit): Boolean {
f()
return true
}
Conclusion
With the when
expression you can make it really easy to create code where you can specify the behaviour through several paths, and where the original Java switch
lack some power.
And if you want to learn how to use Kotlin to develop your own Android Apps, I recommend you take a look at my free training. Reserve your place!