· 3 min read
Variables in Kotlin, differences with Java. var vs val (KAD 02)
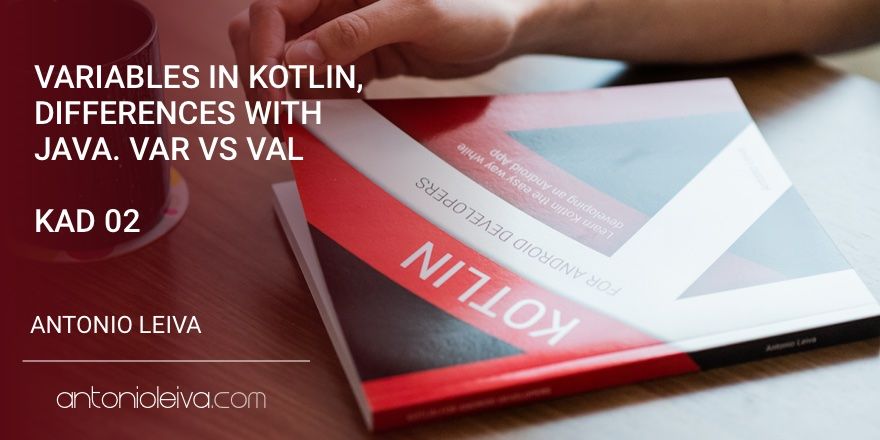
In this second chapter we will see how variables work in Kotlin, what is val and var , and when to use one or the other.
I wanted to start from here, because it will be useful to understand later how to create “fields” in our classes (we will see that they are not exactly fields).
If you prefer the video format, in this one I explain how to create your first project from scratch, and everything about variables:
https://www.youtube.com/watch?v=iRpvLmOxAjw
Variables in Kotlin
Variables in Kotlin allow, as in Java, to assign values that can then be modified and used at different points in our program, as long as they are within the scope in which the code is executed.
But I’m going to focus on the differences we find with Java.
1. The variables can be mutable and immutable
This can also be done in Java (marking variables as final if we don’t want it to be modified), but in Kotlin it is much less verbose and much more used: In Kotlin immutable values are preferred whenever possible.
The fact that most parts of our program are immutable provides lots of benefits, such as a more predictable behaviour and thread safety.
2. Variables are declared using val or var , provided they are immutable or mutable
An interesting thing from Kotlin is that most of the time you won’t need to specify the type of the objects you are working with, as long as the compiler can infer it.
So we just need to write var
or val depending on the type of variable we want to generate, and the type can normally be inferred. We can always specify a type explicitly.
Some examples:
var x = 7
var y: String = "my String"
var z = View(this)
Spoiler: As you see, you do not need to use new to create a new instance of an object.
3. Type casting is done automatically
Whenever the compiler is able to detect that there is no other possible option, the casting will be done automatically. Awesome!
val z: View = findViewById(R.id.my_view)
if (z is TextView) {
z.text = "I've been casted!"
}
Did you see that I did not call
setText()
? I will explain it in next articles!
4. In Kotlin everything is an object
There are no basic types, and there is no void
. If something does not return anything, it actually returns the Unit
object. Most of the time it can be omitted, but it is there, lurking.
Therefore, all these variables are objects:
val x: Int = 20
val y: Double = 21.5
val z: Unit = Unit
5. Simpler numerical types can not be assigned to more complex types
For example, an integer can not be assigned to a long variable. This does not compile:
val x: Int = 20
val y: Long = x
You need to do an explicit casting:
val x: Int = 20
val y: Long = x.toLong()
Conclusion
These are some of the most outstanding differences you can find between variables in Java and Kotlin. In general, variables in Kotlin provide much more flexibility, safety (due to the convention of using val whenever possible) and cleaner, more concise code.
Any doubts? Get ready for the next article!
And if you want to learn how to use Kotlin to develop your own Android Apps, I recommend you take a look at my free training. Reserve your place!