· 3 min read
SwipeRefreshLayout: How to use
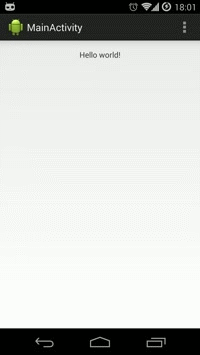
Last support library update has included an interesting and unexpected layout: SwipeRefresLayout. It is a standard way to implement the common Pull to Refresh pattern in Android.
Using this new layout is really easy, but here it is a simple guide to make it work in a few seconds.
What do you need to know?
Almost nothing. Swipe refresh layout is a ViewGroup with the particularity that it can only hold one scrollable view as a children. It is basically a layout decorator that manages touch events and shows an indeterminate progress animation below the action bar when the user swipes down. The effect is similar to the one seen in Google Now app.
Methods are quite few:
- setOnRefreshListener(OnRefreshListener): adds a listener to let other parts of the code know when refreshing begins.
- setRefreshing(boolean): enables or disables progress visibility.
- isRefreshing(): checks whether the view is refreshing.
- setColorScheme(): it receive four different colors that will be used to colorize the animation.
SwipeRefreshLayout: The layout
As said before, you only need to decorate the swipable content (probably the whole layout) with this new layout. This view must be scrollable, such a ScrollView or a ListView. As a simple example:
<android.support.v4.widget.SwipeRefreshLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/swipe_container"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="@string/hello_world"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:gravity="center"/>
</ScrollView>
</android.support.v4.widget.SwipeRefreshLayout>
The code
We just need to get the layout, and assign some colors and the listener. The refreshing listener is a post delayed handler.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
swipeLayout = (SwipeRefreshLayout) findViewById(R.id.swipe_container);
swipeLayout.setOnRefreshListener(this);
swipeLayout.setColorScheme(android.R.color.holo_blue_bright,
android.R.color.holo_green_light,
android.R.color.holo_orange_light,
android.R.color.holo_red_light);
}
@Override
public void onRefresh() {
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
swipeLayout.setRefreshing(false);
}
}, 5000);
}
A convenient activity and a trick
In order to simplify the use of this layout, I created a SwipeRefreshActivity which adds this layout to any activity. It can by found at my Github Gist.
As a trick, you may find it interesting to disable manual swipe gesture, maybe temporarily or because you only want to show progress animation programmatically. What you need to do is to use the method setEnabled() and set it to false.
Conclusion
Finally there is a standard way to create this common design pattern, and be sure we’ll begin to see it more and more in future apps and updates. Unfortunately, I didn’t find a way to change the animation to make it similar to other Apps such as Gmail or Google+, but maybe some subclassing and overriding would do the trick. Keep you informed if I get into it.