· 3 min read
Reified Types in Kotlin: how to use the type within a function (KAD 14)
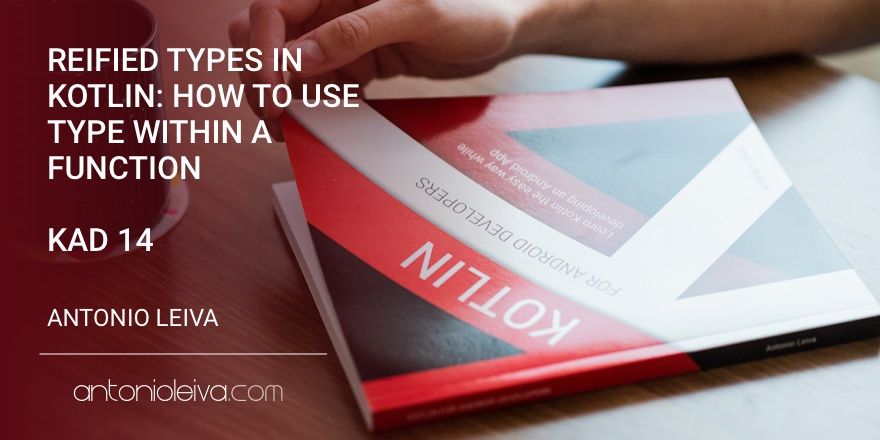
One of the limitations that most frustrates Java developers when using generics is not being able to use the type directly.
Normally this is solved by passing the class as a function parameter, making the code more complex and unattractive.
In Kotlin, thanks to the inline
functions, which we’ve already discussed, we can use reified
types that will allow us to use them within the functions.
What is this for? You’ll see, you’re gonna like it.
If you want to learn inline
and reified
from a different perspective, I also recorded a live video with other samples and approaches:
https://www.youtube.com/watch?v=nxoD5zxkGTs
Reified types
As I commented earlier, by marking a type as reified, we’ll have the ability to use that type within the function.
It is important that the function that uses it is inline, since the code needs to be replaced in the place from which it is run in order to have access to the type. The fact that types can not be used in functions is a limitation of the Java Virtual Machine, and that’s the “trick” to skip that limitation.
Navigating to an Activity
This is the most typical case applied to Android.
In Java, when we call startActivity
, we need to specify the destination class as a parameter.
In Kotlin, we can simplify it by adding the type to the function:
inline fun <reified T : Activity> Activity.startActivity() {
startActivity(Intent(this, T::class.java))
}
Navigating to an Activity
is now as easy as this:
startActivity<DetailActivity>()
FindView with casting
Something that not many Android developers use in Java and that is quite helpful is using generics to return the objects casted to the type of the variable that the result is assigned to.
In Java, you can create a function like this:
public <T extends View> T findView(Activity activity, int id) {
return (T) activity.findViewById(id);
}
And then use it to return the casted object:
TextView textView = Utils.findView(activity, R.id.welcomeMessage);
Something similar can be done with Kotlin, but easier thanks to extension functions:
fun <T : View> Activity.findView(id: Int) = findViewById(id) as T
val textView = activity.findView<TextView>(R.id.welcomeMessage)
But in both cases you’ll find that the compiler can’t be sure that the cast is valid, because it doesn’t have access to the type of T
, so it will show a warning.
With reified types, you can avoid this issue:
inline fun <reified T : View> Activity.findView(id: Int) = findViewById(id) as T
Conclusion
With reified types you can do things that are impossible in Java, and even do some other functions safer.
Now, you can avoid passing an argument of type Class
to your functions.
Besides, thanks to extension functions, you can create new functions over frameworks such as Android, which already use this in some parts of its API (as the startActivity method does).
If you want to learn what Kotlin’s situation is in the market, why you should learn it and the first steps to see how easy it is, I have prepared a free hour and a half training that you can join by clicking here.