· 4 min read
Say goodbye to NullPointerException. Working with nulls in Kotlin (KAD 19)
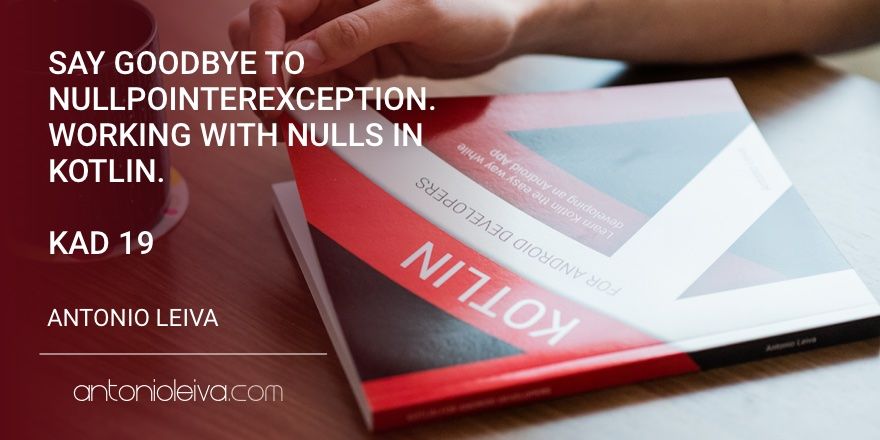
It had taken me too long to write an article to one of the most important parts of Kotlin: the treatment of nullity.
Tony Hoare, the creator of the idea of nulls, calls itself “the billion dollar mistake”. Nulls are one of the most error-prone points you get when you are coding with Java.
If you want to start today, I recommend you take a look at my free training, where you will have an hour and a half of content to know what are your next steps to become an expert in Kotlin.
If you look at your bug manager, I’m sure that +90% of the errors you see are NullPointerException
.
Thanks to Kotlin, you’ll work in a much safer environment (even with Java libraries), which will minimize these problems.
Nulls in Kotlin
Nulls in Kotlin don’t exist until you say otherwise.
That is, no variable, by default, can be set to null
. Remember that in Kotlin all types are objects.
Therefore, this won’t compile:
val x: Int = null
If you want a variable to accept nulls, you have to mark the type with a ?
:
val x: Int? = null
Compile-time check
But, from that point on, the compiler will force you to check the null before doing anything with the variable. This ensures that a NullPointerException
doesn’t occur.
For example:
val y = x.toDouble()
It won’t compile, if you don’t check first if it’s null:
if (x != null) {
val y = x.toDouble()
}
Secure access expression
There is an easier way to represent the previous example, which is to use a ?
before the .
when calling a method.
If the variable isn’t null, it’ll execute the operation. Otherwise, it won’t do anything:
val y = x?.toDouble()
In this case, if x
is null, then the expression will return null as well. So y
will be of type Double?
.
The Elvis operator
But what if we don’t want to have a nullable variable as a result of the operation? The Elvis operator (?:
) allows us to return a value in that case:
val y = x?.toDouble() ?: 0.0
This code would be equivalent to:
val y = if (x != null) {
x.toDouble()
} else {
0.0
}
Spoiler: As you see, most of the sentences in Kotlin are in turn expressions. For example, you can assign the result of an
if
to a variable.
Avoiding the null check
There is an operator (!!
) that will avoid the need to check null
if you’re completely sure that a variable will never be null.
In my opinion, there are very few cases where this operator makes sense. There is almost always a better solution.
But you could do the following:
val x: Int? = null
val y = x!!.toDouble()
This would compile and produce a NullPointerException
What I said: be very careful with this operator.
Java support
When we’re working with Java libraries, we may find ourselves facing different situations regarding null checking.
The library is properly annotated
If the @Nullable
and @NotNull
annotations are being used properly, both Java and Android ones, Kotlin will be able to work seamlessly with them and figure out when a variable is null and when not.
Many parts of the Android framework are already annotated correctly, so this is a huge advantage to working with Kotlin.
The library has no annotations
However, if the library isn’t annotated, the types will be marked with a special operator (a unique !
), which means that it’s in our side to decide if a parameter or return value accepts null or not.
If we have access to the source code, it’s best to check if the code we are using allows nullity or not to make this decision.
An example on Android that isn’t annotated is the RecyclerView
support library. When you create an adapter and generate the methods, by default it’ll add an interrogation to the types.
But if you look at the source code, you’ll realize that nothing can be null in the methods you need to override. So you can get rid of all the interrogation signs, and avoid unnecessary null checks.
Conclusion
The NullPointerException
are a nightmare for all Java developers, and surely represent most of the errors that occur in your code.
Reducing your number in Kotlin to near zero is very easy, even when working with libraries and frameworks in Java.
Only this will avoid you hours and hours of unnecessary debugging, plus it’ll make the code much more stable.
And if you want to learn how to use Kotlin to develop your own Android Apps, I recommend you take a look at my free training. Reserve your place!