· 3 min read
How lambdas work in Kotlin. setOnClickListener transformation (KAD 18)
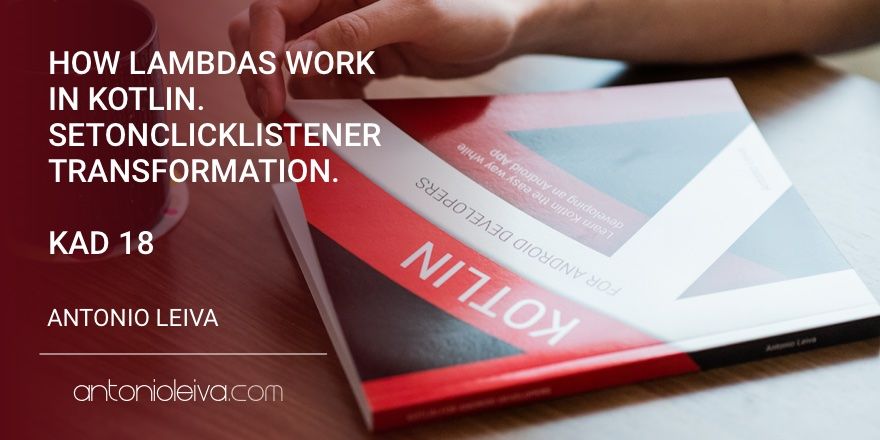
Although I spoke a little about it in another article, I’d like to explain in depth how lambdas work in Kotlin, and how they transform the interfaces with a single method in lambdas when we are working with Java libraries.
In particular I’m going to show you some examples on how to simplify the use of the Android Framework, and we’ll see the setOnClickListener
of the Android views in detail.
Would you like to start today to take the next step? I recommend that you sign up to my free training here.
SetOnClickListener transformation
One of the features I like most about Kotlin is that it simplifies the work with the Android framework thanks to some conventions.
For example, the setOnClickListener
function, which in Java is defined as:
public void setOnClickListener(OnClickListener l) {
...
}
When we use it in Kotlin, it corresponds to the following:
fun setOnClickListener(l: (View) -> Unit)
This saves us the need to have to create an anonymous implementation of the interface, greatly simplifying the initialization of UI components.
Using setOnClickListener. The original way
Following the above, we’ve already saved enough code. This is what we would have if we had to create an anonymous class of OnClickListener
:
view.setOnClickListener(object : View.OnClickListener {
override fun onClick(v: View?) {
toast("Hello")
}
})
But you’ll see that the editor shows you a warning directly, and recommends using the lambda way.
This is the transformation you can do:
view.setOnClickListener({ v -> toast("Hello") })
Easier, right? But this can be simplified even more.
If the function’s last parameter is a function, it can go outside the parentheses
Therefore, we can extract the listener as follows:
view.setOnClickListener() { v -> toast("Hello") }
If we had more parameters, the rest of the parameters would go inside the parentheses, even if these were functions. Only the last parameter can be extracted.
If a function has only one parameter, and this is a function, the parentheses can be deleted
Instead of having empty parentheses, we can better delete them:
view.setOnClickListener { v -> toast("Hello") }
This comes great for building code blocks. In this way we can define DSLs that model our own language.
A very typical example is the Kotlin reference page, where they build a DSL to create HTML by code.
If you don’t use the parameter of a lambda, you can remove the left side of the function
This is true if you only have one parameter. The view (v
) isn’t being used, so we can remove it:
view.setOnClickListener { toast("Hello") }
In addition, in functions that only receive a parameter, instead of defining the left side, we could use the reserved word it
, saving some characters.
For example, if we used the view to pass it to another method:
view.setOnClickListener { v -> doSomething(v) }
We have the option to simply use it
:
view.setOnClickListener { doSomething(it) }
Your friends, the Lambdas
You see that the code difference is quite important. And not only for the characters you save (about 70%), but it greatly improves readability.
Instead of having to skip all the code that does nothing to find the useful part, we just write what really matters.
Cheer up and come to my free training. I will tell you everything you need to learn about how to create your own Android Apps in Kotlin from scratch. Discover everything about the language of the future.