· 3 min read
Kotlin integrations with Android SDK (KAD 05)
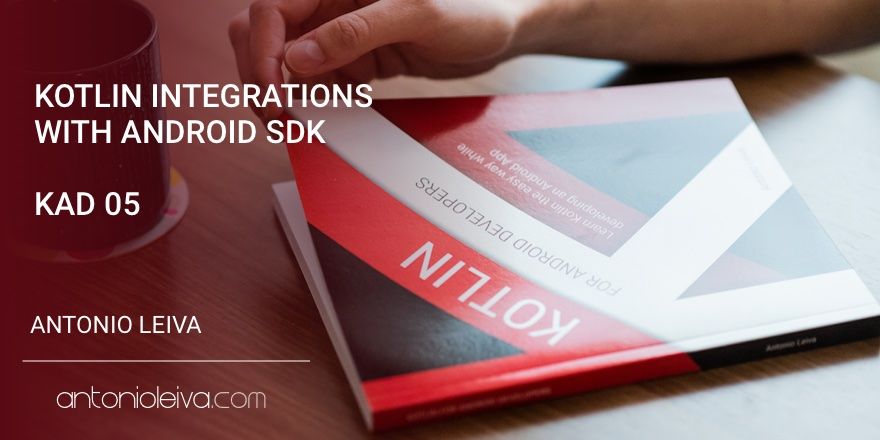
Kotlin not only simplifies the code you create on this language, but also the Java code you use from Kotlin.
How does this work? It simply uses some generic structures and give them a more “Kotlin” feel.
In this article, you’ll see some examples, and how it improves code homogeneity. It also prevents part of the boilerplate involved in Java code.
Kotlin integrations with Android SDK
As Android framework is basically a Java library, everything we mentioned before about Java applies perfectly to Android.
Let’s see some examples
getters and setters are mapped to properties
We’ve already seen this in previous articles. This is the explanation I promised.
As we talked, Kotlin uses properties instead of fields + getters + setters, and the way to assign values and get them is the same we’d use in a public field in Java.
But as we know, there’s a code being executed that can be a simple assignment, or the custom operation we implement.
The rule here is that any setX
and getX
found in Java will be mapped to an x
in Kotlin.
For instance, if you are using a TextView
, you can set the text using a property-like sentence:
val textView: TextView = ...
textView.text = "My Text"
And this applies to any classes, of course. You can access to applicationContext
or layoutInflater
in an Activity (for example) the same way:
layoutInflater.inflate(R.layout.view_item, parent)
val hello = applicationContext.getString(R.string.hello)
If you are worried about performance, in fact these this will keep mapping to the original getters and setters, so it’s the same as calling them directly.
Interfaces with one method are mapped to lambdas
This is awesome. Aren’t you tired of creating anonymous classes for any simple things in Android? With Kotlin, you can prevent doing this for many Android listeners.
The condition is this: it needs to be an interface with a single method. The more typical example is setOnClickListener
from View
class.
You can do something as simple as this:
view.setOnClickListener { navigateToNextActivity() }
Saw how easy?
If you need the view that is returned from the original interface, you can also recover it:
view.setOnClickListener { v -> navigateWithView(v) }
Spoiler: What’s this weird structure? It’s called lambda, and it’s a way to represent functions where the input parameters are defined at the left side of the arrow. The right side of the arrow is the body of the function, and the last line is the result. We’ll see some about it in future articles.
Conclusion
It’s that easy to integrate other Java libraries, and the Android framework in particular, in Kotlin projects. The good part is that it also looks like Kotlin, which is helpful both because the code is simpler and because all your code will feel like Kotlin.
If you want to learn more about Kotlin, join the list and you’ll receive them right in your inbox.