· 3 min read
Kotlin for Android (IV): Custom Views and Android Extensions
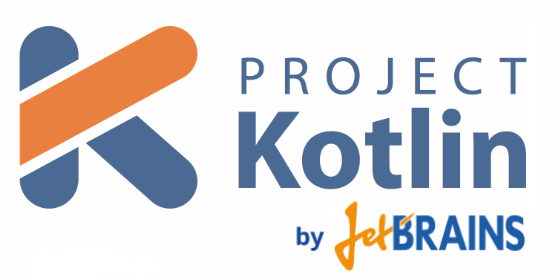
After reading what extension functions and default values can do for you, you might be wondering what´s next. As we talked in first article about Kotlin, this language makes Android development much simpler and there are still some more things I´d like to talk about.
Custom Views
Kotlin, by default, only uses one constructor per class. This is usually enough, because using optional parameters we can create as many variations of the constructor as we may need. Here it is an example:
class MyClass(param: Int, optParam1: String = "", optParam2: Int = 1) {
init {
// Initialization code
}
}
With a unique constructor, we now have four ways to create this class:
val myClass1 = MyClass(1)
val myClass2 = MyClass(1, "hello")
val myClass3 = MyClass(param = 1, optParam2 = 4)
val myClass4 = MyClass(1, "hello", 4)
As you see, we get a whole bunch of combinations just by using optional parameters. But this leads to a problem if we are trying to create an Android custom view by extending one of the regular views. Custom views need to override more than one constructor to work properly. Luckily, we have a way to declare more constructors in way similar to what we do in Java. This is an example of an ImageView which preservers a squared ratio:
class SquareImageView : ImageView {
constructor(context: Context) : super(context)
constructor(context: Context, attrs: AttributeSet) : super(context, attrs)
constructor(context: Context, attrs: AttributeSet, defStyleAttr: Int) : super(context, attrs, defStyleAttr)
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec)
val width = getMeasuredWidth()
setMeasuredDimension(width, width)
}
}
Quite simple. It could probably be less verbose, but at least we have a way to do it.
Kotlin Android Extensions
If you didn’t know about Kotlin Android Extensions, you’re gonna love them. They will help us Android developers to access to the views declared in an XML in a much easier way. Some of you will remember Butterknife when you see it, but it´s even simpler to use.
Kotlin Android Extensions is basically a view binder that will let you use your XML views in your code by just using their id. It will automatically create properties for them without using any external annotation or findViewById methods.
To start using it, you´ll need to include the new plugin into the build.gradle:
apply plugin: 'com.android.application'
apply plugin: 'kotlin-android'
apply plugin: 'kotlin-android-extensions'
...
buildscript {
repositories {
jcenter()
}
dependencies {
classpath "org.jetbrains.kotlin:kotlin-android-extensions:$kotlin_version"
}
}
Imagine you have declared the next layout, called activity_main.xml:
<FrameLayout
xmlns:android="..."
android:id="@+id/frameLayout"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/welcomeText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</FrameLayout>
If you want to use these views in your activity, the only thing you need to do is importing the synthetic properties for that xml:
import kotlinx.android.synthetic.main.<xml_name>.*
In our case, it will be just activity_main:
import kotlinx.android.synthetic.main.activity_main.*
Now you can access your views by using its id:
override fun onCreate(savedInstanceState: Bundle?) {
super<BaseActivity>.onCreate(savedInstanceState)
setContentView(R.id.main)
frameLayout.setVisibility(View.VISIBLE)
welcomeText.setText("I´m a welcome text!!")
}
Conclusion
What it´s really promising about these two features is that it´s clear that the Kotlin team is very interested in making Android developers lives easier. They also released a library called Anko, a DSL to create Android layouts from Kotlin files. I´m not using its main functionality yet, but you can use it to simplify your code when dealing with Android views, and I have some examples of this in the Kotlin project I pushed to Github. You can take a look to see this and many other things.
Next article will cover the use of lambda expressions and how they can help us simplify our code and extend the language. Really interesting one! To me, the most powerful aspect of Kotlin when compared with Java 1.7.