· 3 min read
Design Support Library (III): Coordinator Layout
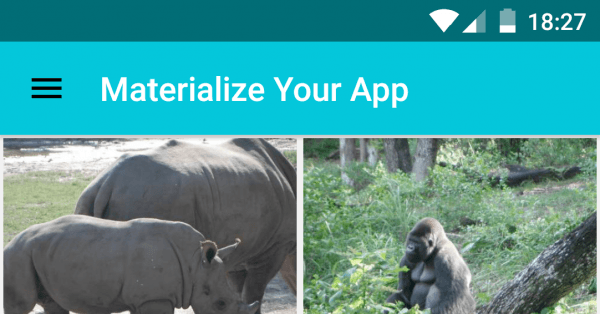
Today, I´d like to take a look to the Coordinator Layout and some basic ideas about App Bar Layout. Together, they can do some nice animated effects. In this occasion, we´ll see how to hide the toolbar while we start scrolling down, and then show it again as soon as we start scrolling down.
We´ll also see how to make a Floating Action Button animate to leave enough space for a Snack Bar to show.
Coordinator Layout
The Coordinator Layout is one of the new powerful layouts introduced in the new design support library. We already talked about the Navigation View and the Floating Action Button, but now it´s time to put everything to work together and coordinate their animations.
That´s what this new layout is all about: based on a set of rules defined in Behaviors, we can define how views inside the Coordinator Layout relate each other and what can be expected when one of them changes. I´ll talk about behaviours in a future post, but they identify which views another view depend on, and how they behave when any of these views changes.
App Bar Layout
This layout must surround the views that conform the toolbar to make them animate the way we want based on a scrolling view. We can define the scrolling view in XML using this attribute:
app:layout_behavior="@string/appbar_scrolling_view_behavior"
This will add a behavior to the view, which can be listened by the AppBarLayout
to animate its children.
For example, in MaterializeYourApp example, I´m using a RecyclerView
in main activity. This is how the XML looks like:
<android.support.v7.widget.RecyclerView
android:id="@+id/recycler"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"/>
By using some scroll flags, we can specify the behaviour of the views inside the layout. We have these different options:
- scroll: This means it will scroll while scrolling the targeted view (our recycler view in this case).
- enterAlways: When we scroll up, the view will immediately reappear.
- enterAlwaysCollapsed: if the view has a collapsed mode, it will reappear collapsed when scrolling up.
- exitUntilCollapsed: it won´t exit from the screen until the view is collapsed.
I´ll review some of these flags in next articles, but for the main activity, we just want it to scroll with the scrolling view and to re-enter as soon as we scroll up. So that´s the combination the Toolbar
will use:
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light"
app:layout_scrollFlags="scroll|enterAlways" />
This is the important line:
app:layout_scrollFlags="scroll|enterAlways" />
Coordinator Layout, Floating Action Bar and Snack Bar
This is almost for free, but it´s interesting to know that FloatingActionButton
behavior will pay attention to Snack Bar views and move to leave them enough space to paint. The only requirements are that the FAB is inside a Coordinator Layout and that we attach the SnackBar
to it:
findViewById(R.id.fab).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Snackbar.make(content, "FAB Clicked", Snackbar.LENGTH_SHORT).show();
}
});
The content
view in this case is the CoordinatorLayout
, so when the FAB is clicked, the SnackBar
appears and the FAB animates with it.
Conclusion
Thanks to CoordinatorLayout
and AppBarLayout
you are starting to discover the amazing animations you can get without hardly any code. This is going to be bigger when you are able to create more complex toolbars which animate in many different ways, really useful to create lively detail screens. But this will have to wait to the next article, where I’ll talk about Collapsing Toolbar Layout. In the meanwhile, you can check the working code in MaterializeYourApp repo example.