· 3 min read
Classes in Kotlin: More power with less effort (KAD 03)
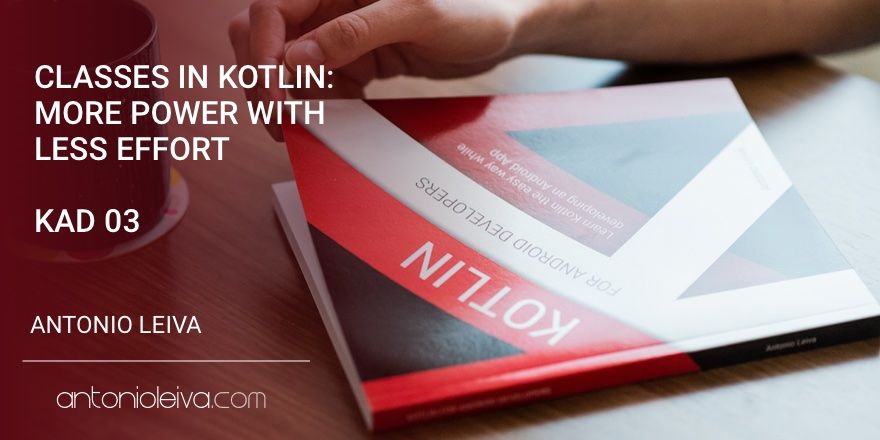
Classes in Kotlin are as simple as possible so that you can express the maximum amount of logic with the less code possible.
I’ll show quickly how you can start writing Kotlin classes, and the differences with Java classes.
1. Declare the class
class Person
It’s as easy as using the reserved word class and the name of the clase. You don’t need to use braces if the class doesn’t contain any code.
Spoiler: Have you seen it’s not using public visibility modifier? That’s because everything is public in Kotlin by default.
2. Add some properties
Fields don’t exist in Kotlin (or at least you can’t declare them). A class has properties.
To put it simple, a property substitutes a Java field + getter + setter. So let’s declare a couple of properties for our class:
class Person {
var name = "Name"
var surname = "Surname"
}
If you want a custom setter, of course you can declare it:
var name = "Name"
set(value) {
name = "Name: $value"
}
Spoiler: as you can see, you can use variables directly into Strings (😱), without using the typical
String.format
Have you realized the amount of code you’ll save with this? All the setters and getters are immediately blown out.
3. Add a constructor
By default, you only need a constructor per class. You can add several, but you’ll see this use in a future article.
As there’s only one constructor, the way to write it can be simplified a lot too:
class Person(val name: String, val surname: String)
What happened here?
- The properties disappeared! In fact, they’re still there. But if the constructor arguments are annotated with var or val, the properties are generated inline.
- The constructor is written just after the definition of the class. You don’t need to create an extra method and assign the values of the properties.
4. You can now create functions inside the class
And use the properties inside them as you wish:
class Person(val name: String, val surname: String) {
fun getFullName() = "$name $surname"
}
As the example above shows, functions can be written in a contracted way when a value is directly assigned. But there still exists the old “Java” way:
fun getFullName(): String {
return "$name $surname"
}
In this case, you need to define the return type.
5. Everything in Kotlin is closed by default
So it can’t be extended, and children (in case a class can be extended) can’t override its functions, unless it’s indicated with the reserved word open:
open class Person(val name: String, val surname: String)
class Cop(surname: String) : Person("Mr", surname)
See how the parent constructor is called. Simple and clean!
Conclusion
In this article, you’ve seen some of the differences between Java and Kotlin classes. If you want to know more about them, I recommend you to subscribe to receive the rest of the articles I’ve prepared for you.
If all this passionate you as to me, I encourage you to sign up for my free training where I will tell you everything you need to learn about how to create your own Android Apps in Kotlin from scratch.